不是很会拍夜景,另外,光圈不够大,拍不到我想要的效果。
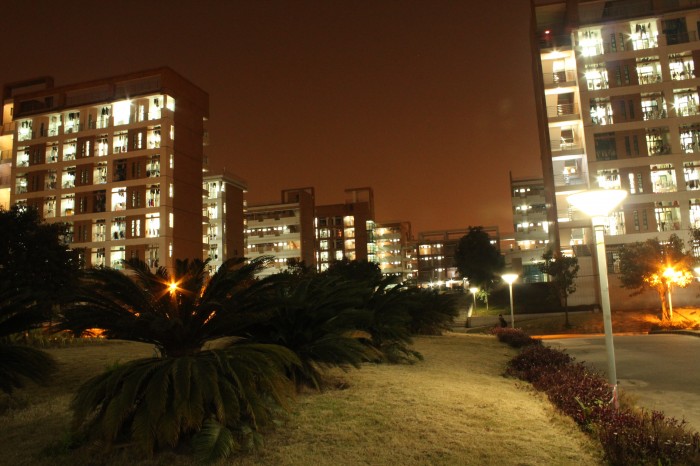
引用原题:
3. Write a problem to keep track of streaming price data coming from the stock market.
The input data will consist of one transaction per line containing separated values
with the following fields: TimeStamp, Stock ID, Price per volume and Volume as:
YYYY MM DD HH MM SS StockID Price Volume
The output of the program will be sorted by the stockID, with one record per line that
summarizes the data as follows. For each day, the program will output one line that
prints:
1. The time stamp at the end of the period
2. The current price per the volume at the end of a day (curPrice)
3. The average price per the volume for a day (avgPrice)
4. The lowest price per the volume for a day (lowPrice)
5. The highest price per the volume for a day (hightPrice)
6. The total volume. (TVolume)
YYYY MM DD HH MM SS StockID curPrice avgPrice lowPrice hightPrice TVolume
Be noted that the input is not sorted so you do not know exactly how the input stream
arrives. The last line of input containing the number -1 signals the end of the input.
Example:
Input:
2010 01 30 10 00 00 2 3.72 10000
2010 01 31 12 28 00 1 3.78 500000
2010 01 30 10 00 00 1 3.74 100000
2010 01 30 10 00 05 1 3.77 50000
-1
Output:
2010 01 30 10 00 05 1 3.77 3.75 3.74 3.77 150000
2010 01 30 10 00 00 2 3.72 3.72 3.72 3.72 10000
2010 01 31 12 28 00 1 3.78 3.78 3.78 3.78 500000
2010 01 31 00 00 00 2 0.00 0.00 0.00 0.00 0
#include <stdio.h> #include <stdlib.h> #include <string.h> #include <vector> #include <algorithm> using namespace std; /* 这里有个有趣的问题,如果不加一点点(例如.00001), * 则会把3.55变成了3.54来输出,不知为什么。 * Call me Xiaoxia */ #define YES(a) (((int)((a+.00001)*100))/100.0) struct stock{ int year, mon, day; int hour, min, sec; unsigned int totsec, totsec2; int id; float price; int volume; }; /* first key: date second key: id third key: time */ bool cmp(stock* const& a, stock* const &b) { if( a->totsec < b->totsec ) return true; if( a->totsec > b->totsec ) return false; if( a->id < b->id ) return true; if( a->id > b->id ) return false; if( a->totsec2 < b->totsec2 ) return true; return false; } stock* s; vector<stock*> v; char vid[1000000]; int maxvid=0; /* display stock id from a to b */ void skipid(int a, int b, stock* olds) { for(int i=a+1; i<=b-1; i++) if(vid[i]) printf("%d %02d %02d %02d %02d %02d %d %.2f %.2f %.2f %.2f %u\n", olds->year, olds->mon, olds->day, 0, 0, 0, i, .0, .0, .0, .0, 0); } int main(int argc, char **argv) { memset(vid, 0, sizeof(vid)); for(;;){ s = new stock; scanf("%d", &s->year); if(s->year==-1) break; /* memory leaks i dont care */ scanf("%d %d %d %d %d %d %f %d", &s->mon, &s->day, &s->hour, &s->min, &s->sec, &s->id, &s->price, &s->volume); s->totsec = (s->year<<16) | (s->mon<<8) | (s->day); /* date */ s->totsec2 = (s->hour<<16) | (s->min<<8) | (s->sec); /* time */ v.push_back(s); vid[s->id] = 1; if(s->id>maxvid) maxvid = s->id; } sort(v.begin(), v.end(), cmp); unsigned int date = v[0]->totsec; stock* olds=v[0]; unsigned int lastid = 0; for(int i=0; i<v.size(); ){ s = v[i]; if(s->totsec!=olds->totsec){ skipid(lastid, maxvid+1, olds); lastid = 0; } skipid(lastid, s->id, s); olds = s; float highprice=0.0, lowprice=99999.0, totprice=0.0; int totvol=0, cnt=0; /* find all the same id stocks of the same day */ for(int j=s->id, dat=s->totsec; s && j==s->id && dat==s->totsec; i++, olds=s, s=i<v.size()?v[i]:0, cnt++){ totprice += s->price; totvol += s->volume; if(s->price < lowprice) lowprice = s->price; if(s->price > highprice) highprice = s->price; } printf("%d %02d %02d %02d %02d %02d %d %.2f %.2f %.2f %.2f %u\n", olds->year, olds->mon, olds->day, olds->hour, olds->min, olds->sec, olds->id, olds->price, YES(totprice/cnt), lowprice, highprice, totvol); lastid = olds->id; if(i>v.size()) break; } skipid(lastid, maxvid+1, olds); return 0; }
可以用STL吗??Orz
当然可以!
浮点数的精度问题吧?换double试试?
难道double之后就不会把3.55变成3.54了?
3.55转换为二进制时,位的长度可能超过FLOAT的32位,因此会被舍掉一部分。得到的结果比3。55小,转换为10进制就是3。54
非常有深度的解释!!!赞!!!学习了~